Python: Cycle For
Instructions
Loading...
Your exercise will be checked with these tests:
from index import filter_string
def test1():
text = "If I look forward I am win"
assert filter_string(text, "z") == "If I look forward I am win"
assert filter_string(text, "I") == "f look forward am wn"
assert filter_string("zz zorro", "z") == " orro"
Teacher's solution will be available in:
20:00
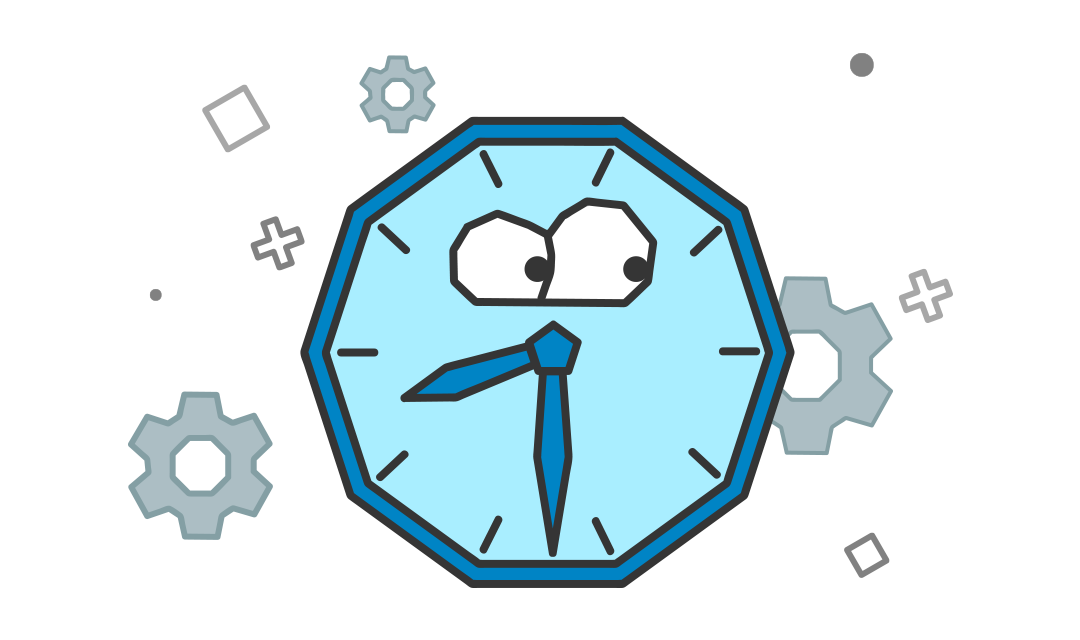