Python: Function Parameters
Instructions
Loading...
Your exercise will be checked with these tests:
import index
def test():
assert index.truncate("hexlet", 2) == "he..."
assert index.truncate("it works!", 4) == "it w..."
Teacher's solution will be available in:
20:00
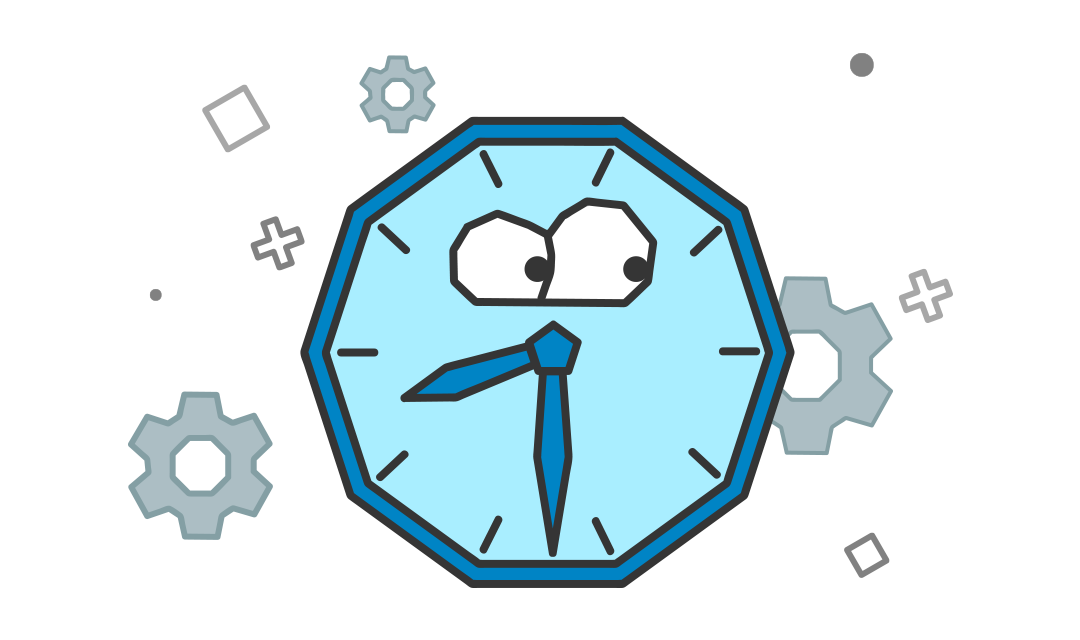