JavaScript: Magic numbers
Instructions
Loading...
Your exercise will be checked with these tests:
// @ts-check
import { expect, test, vi } from 'vitest'
test('hello world', async () => {
const consoleLogSpy = vi.spyOn(console, 'log').mockImplementation(() => { });
await import('./index.js')
const firstArg = consoleLogSpy.mock.calls.join('\n');
expect(firstArg).toBe('King Balon the 6th has 102 rooms.')
})
Teacher's solution will be available in:
20:00
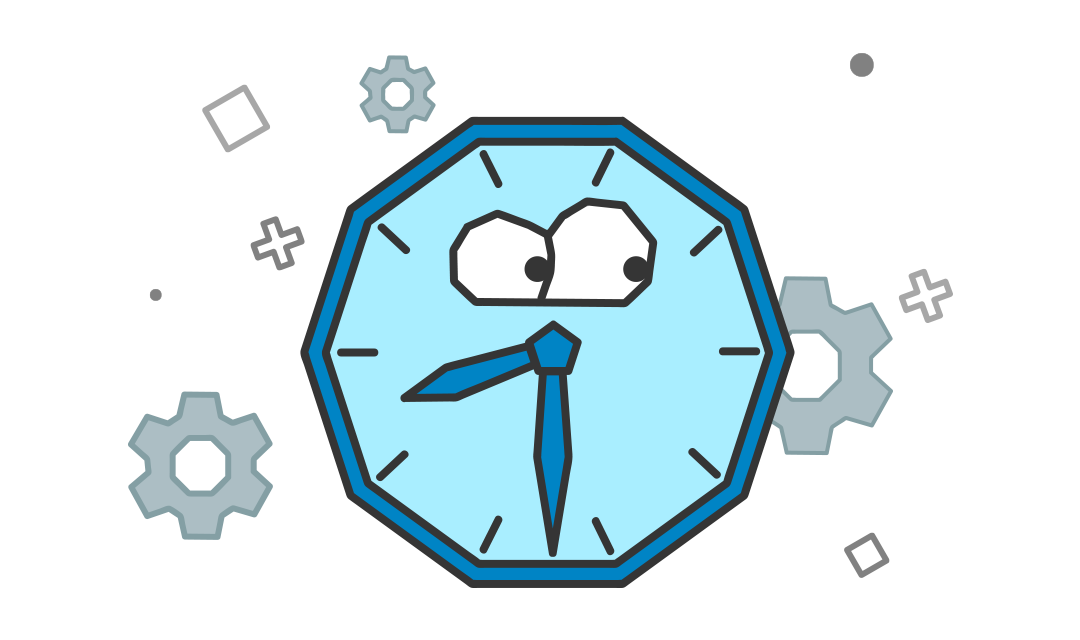