JavaScript: Function parameters
Instructions
Loading...
Your exercise will be checked with these tests:
// @ts-check
import { expect, test } from 'vitest';
import f from './index.js';
test('test', () => {
expect(f('текст', 3)).toBe('тек...');
expect(f('и пошла вода', 5)).toBe('и пош...');
});
Teacher's solution will be available in:
20:00
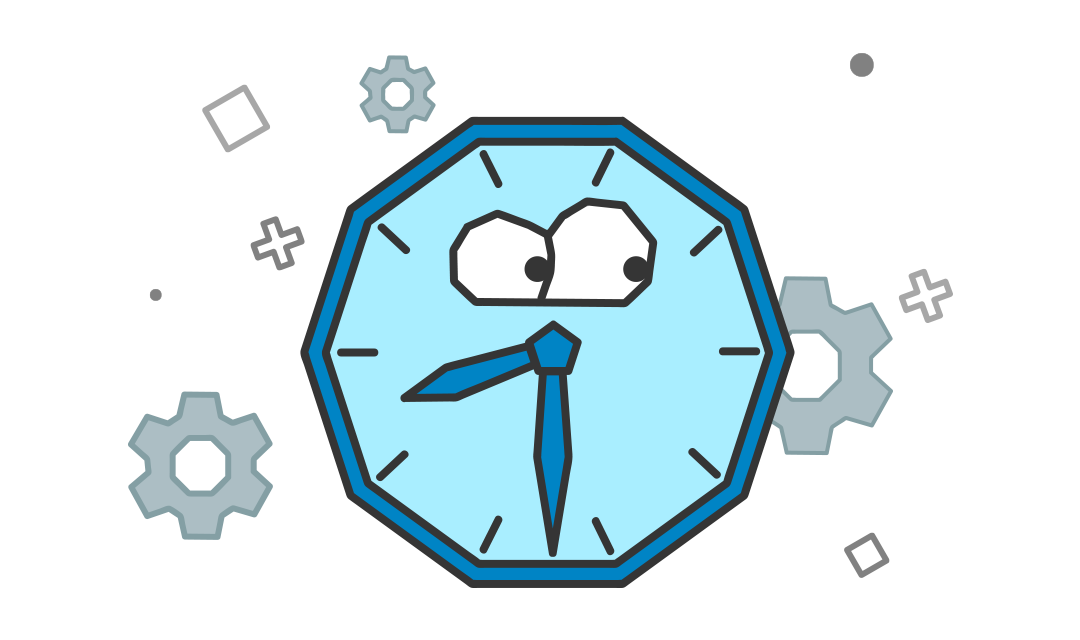